Scheduled Work
Type | scheduledwork |
Datastore Type | platform |
Supports | authorities, permissions, transfer |
Configuration
what frequency and what settings are provided for their described actions. You can adjust this confirmation at any time and supply it when you create, update or query for scheduled work items.
The following table shows the configuration properties:
Property | Type | Default | Read-Only | Description |
---|---|---|---|---|
triggerConfig | object | An object that describes the frequency of the trigger. { "frequency": "{frequency}", "day": "{day}", "hour": "{hour}", "timezone": "{timezone}" } |
Working with Scheduled Work Items
If you have platform manager rights, you will be able to work with scheduled work items from either the Cloud CMS Administration Console or programatically.
Cloud CMS Administration Console
On the left-hand side, you will see a Scheduled Work page.
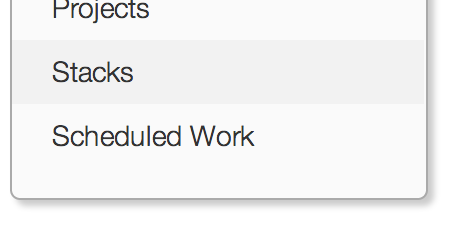
From this page, you can list or query for existing scheduled work items. You can also edit existing entries or create brand new scheduled work items.
To create a scheduled work item, click Create. You'll see a form like this:
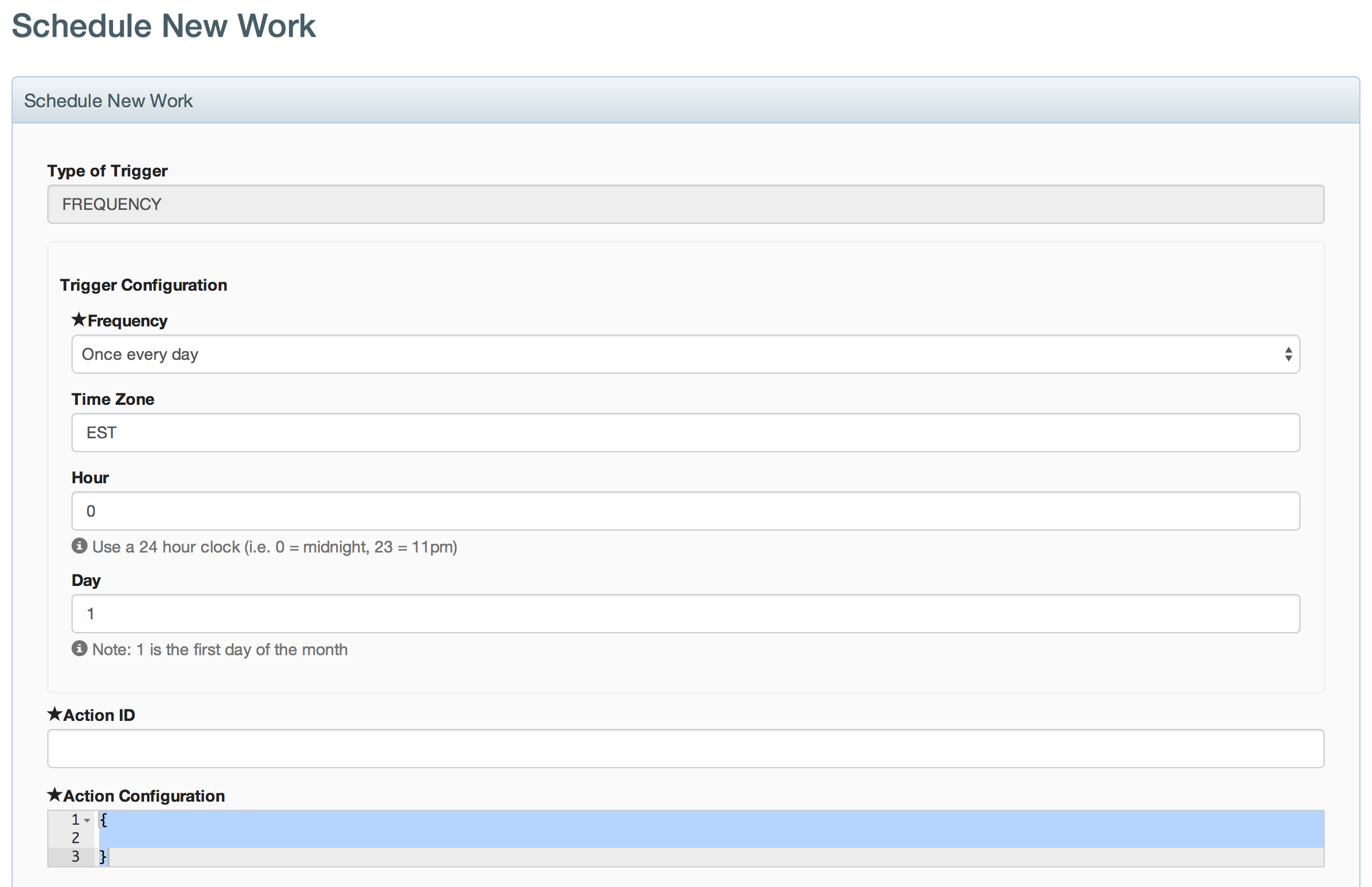
You can use this form to set all of the properties of your work item. For information on configuring the action that the scheduled work item triggers, please see below.
API
You can create a scheduled work item by simply providing the JSON to configure it:
// the platform
var platform = ...;
// create the scheduled work item
// this runs at 5pm every day (EST)
platform.createScheduledWorkItem({
"triggerConfig": {
"frequency": "ONCE_EVERY_DAY",
"hour": 17,
"timezone": "EST"
},
"actionId": "email",
"actionConfig": {
"to": "joe@bloggs.com",
"body": "Hi Joe!"
}
}).then(function() {
// all done
});
To update, simply adjust it's configuration as you see fit. Cloud CMS will automatically adjust any live triggers that are running across the cluster according to your definition.
// assume we have a scheduled work item
var scheduledWorkItem = ...;
// update the schedule
// run at 1pm GMT in the middle of the month
scheduledWorkItem["triggerConfig"]["frequency"] = "ONCE_EVERY_MONTH";
scheduledWorkItem["triggerConfig"]["day"] = 15;
scheduledWorkItem["triggerConfig"]["hour"] = 13;
scheduledWorkItem["triggerConfig"]["timezone"] = "GMT";
// and change the email
scheduledWorkItem["actionConfig"]["body"] = "Hello Joe!";
scheduledWorkItem.update();
And, of course, you can delete scheduled work items.
// assume we have a scheduled work item
var scheduledWorkItem = ...;
// delete it
scheduledWorkItem.del();
You can also list and query for scheduled work items using their JSON properties. Just like anything else in Cloud CMS, lists and queries support pagination, sorting and deeply nested property lookups.
Here is an example of querying for scheduled work items:
// the platform
var platform = ...;
// find all scheduled work items that are running monthly on the 3rd day of the month
platform.queryScheduledWorkItems({
"triggerConfig.frequency": "ONCE_EVERY_MONTH",
"triggerConfig.day": 3
}).each(function() {
console.log("Found: " + this._doc);
});