HTTP Deployment Handler
Handles the deployment of one or more Deployment Packages to a custom HTTP endpoint.
The custom HTTP endpoint is described by:
url
statusUrl
username
password
Custom HTTP endpoints are useful for a large variety of purposes, including writing data into custom databases, synchronizing content from Cloud CMS with existing Content Management products, hooking into custom logic for CDN or cache invalidation and much more.
Configuration
{
"url": "{url}",
"statusUrl": "{statusUrl}",
"username": "{username}",
"password": "{password}"
}
Where url
is the remote address of the server and username
and password
describe the credentials to be applied using Basic Authentication. The statusUrl
is optional - it enables Cloud CMS to poll your endpoint for completion.
How it works
The remote HTTP endpoint must be a POST handler. All POSTs to the endpoint will be multipart/form-data
and will contain the following parts:
package
archive-{guid1}
archive-{guid2}
...
There will be at least 2 parts (package
and archive-{guid1}
) where guid1
is the ID of the archive that contains the JSON and binaries being deployed. A deployment package may also have multiple archives in which case there will be more parts. Your custom HTTP endpoint should consider the prefixes of the incoming parts to determine how each is applicable.
The package
part provides the JSON for the deployment package.
The HTTP endpoint should acknowledge receipt of the deployment package by returning a 200 and a JSON payload that contains an ID for the deployment package on the endpoint. It may look like this:
{
"_doc": "{remoteDeploymentPackageId}"
}
This _doc
, for all intents and purposes, serves as a job ID or a task ID for the background processing that is now occurring against the deployment package on the remote HTTP server.
The statusUrl
property is optional. If it is provided, Cloud CMS will poll the HTTP endpoint using the aforementioned _doc
to check on the status of the deployment package.
If you do choose to provide statusUrl
, then it should point to a GET
method that returns the deployment package JSON. This JSON should more or less look like this:
{
"_doc": "",
"state": "PENDING|PROCESSING|SUCCESS|ERROR",
"operation": "DEPLOY|UNDEPLOY",
"retain": true|false,
"records": [{
"_doc": "",
"state": "NONE|DIRTY|PROCESSING|FINISHED|ERROR",
"operation": "DEPLOY|UNDEPLOY",
"ref": "",
"success": true|false,
"message": ""
}, ...]
}
The state
of the deployment package indicates whether the HTTP endpoint has finished processing all of the records contained within it. When it finishes, it should either flip to SUCCESS
or ERROR
. Further, each individual deployment record should have its state adjusted to FINISHED
or ERROR
. Further, the success
field should be tripped to either true
or false
. Any deployment records that fail should have their message
set so that meaningful information can be conveyed back to Cloud CMS about what went wrong.
The statusUrl
value is allowed to use a special id
field to fill in the _doc
of the deployment record as acquired from the initial push. You can populate this using handlebars, like this:
http://deployer.mywebsite.com/status/{{id}}
Or:
http://deployer.mywebsite.com/status?id={{id}}
Example Configuration
{
"url": "http://deployer.mywebsite.com/push",
"statusUrl": "http://deployer.mywebsite.com/status/"
"username": "inigo",
"password": "montoya"
}
Building your own HTTP Deployment Receiver
We encourage you to go on and build some really cool and interesting Cloud CMS Deployment Receivers. To help you get started, we provide a Node.js-based custom HTTP Deployment Receiver that shows off what is possible. It is available in our SDK, here:
https://github.com/gitana/sdk/tree/master/http-deployment-receiver
To configure this within your own Cloud CMS environment, just add an http
Deployment Target and configure it a bit like this:
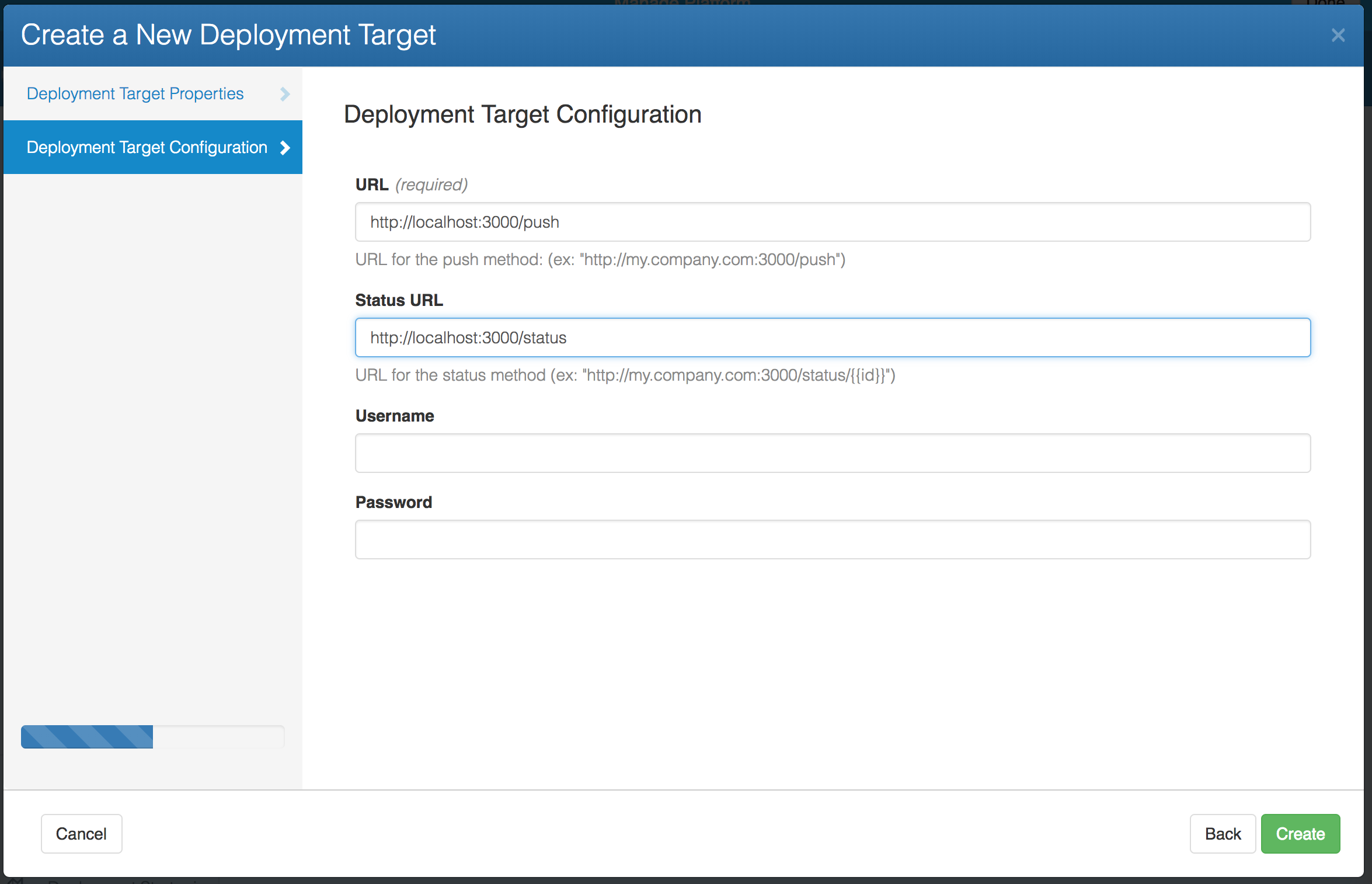
This Custom HTTP Deployment Receiver uses a small library which is available here:
https://github.com/gitana/cloudcms-http-deployment-receiver
We encourage you to take a closer look at this library. It may be give you a head start in terms of building out your own custom HTTP Deployment Receivers. At the very least, it should serve as a useful technical reference to compare your own implementations against.
When testing your receiver, you need to ensure that the endpoint is accessible to the outside world, or at the least accessible to the API. On premise users using the API in a docker container hosted on a Mac or Windows PC can access receiver services hosted on the same machine by using the host.docker.internal
address. So, for example, when creating your deployment receiver in cloudcms to connect to a local server bound to port 3000, you would configure to the url parameter as http://host.docker.internal:3000
.