Scripts
Scripts are content nodes with a default attachment of type application/javascript
.
As with all behaviors, script nodes must implement the f:behavior
feature. They must be bound to a node upon which to act (either a definition node or a content instance) using a a:has_behavior
association.
Script Interfaces
If you elect to write scripts to implement custom behaviors, the following signatures can be used inside of your JavaScript:
Association Policies
- function beforeAssociate(association)
- function afterAssociate(association)
- function beforeUnassociate(association)
- function afterUnassociate(association)
Attachment Policies
- function beforeReadAttachment(node, attachmentId)
- function afterReadAttachment(node, attachmentId)
- function beforeCreateUpdateAttachment(node, attachmentId, contentType, filename)
- function afterCreateUpdateAttachment(node, Attachment attachment)
- function beforeDeleteAttachment(node, Attachment attachment)
- function afterDeleteAttachment(node, Attachment attachment)
Data List Policies
- function beforeAddListItem(item, list)
- function afterAddListItem(item, list)
- function beforeUpdateListItem(item, list)
- function afterUpdateListItem(item, list)
- function beforeRemoveListItem(item, list)
- function afterRemoveListItem(item, list)
Node Policies
- function afterReadNode(node)
- function beforeCreateNode(node)
- function afterCreateNode(node)
- function beforeUpdateNode(node, originalNode)
- function afterUpdateNode(node, originalNode)
- function beforeDeleteNode(node, originalNode)
- function afterDeleteNode(node)
- function beforeTouchNode(node)
- function afterTouchNode(node)
- function beforeAssociateNode(node, association, associatedNode)
- function afterAssociateNode(node, association, associatedNode)
- function beforeUpdateAssociatedNode(node, association, associatedNode)
- function afterUpdateAssociatedNode(node, association, associatedNode)
- function beforeUnassociateNode(node, association, associatedNode)
- function afterUnassociateNode(node, association, associatedNode)
- function beforeAddFeature(node, FeatureDefinition definition, Object configuration)
- function afterAddFeature(node, FeatureDefinition definition, Object configuration)
- function beforeUpdateFeature(node, FeatureDefinition definition, Object configuration)
- function afterUpdateFeature(node, FeatureDefinition definition, Object configuration)
- function beforeRemoveFeature(node, FeatureDefinition definition, Object configuration)
- function afterRemoveFeature(node, FeatureDefinition definition, Object configuration);
Property Policies
- function beforeCreateProperty(node, name, value)
- function afterCreateProperty(node, name, value)
- function beforeUpdateProperty(node, name, value, originalNode, originalValue)
- function afterUpdateProperty(node, name, value, originalNode, originalValue)
- function beforeDeleteProperty(node, name, value)
- function afterDeleteProperty(node, name, value)
- function beforeTouchProperty(node, name, value, originalValue)
- function afterTouchProperty(node, name, value, originalValue)
Read more about Content Policies within Cloud CMS.
An Example - Script Behavior
In this example, we'll define a behavior that maintains a counter for us. The counter will count the number of times that a document has been updated. We'll then register this behavior so that it triggers whenever content of a particular type is updated.
Define the Behavior using JavaScript
Let's start by creating the behavior. We'll do this using server-side JavaScript. Go to Folders and browse to a folder. Then click on Actions > Create a Text File.
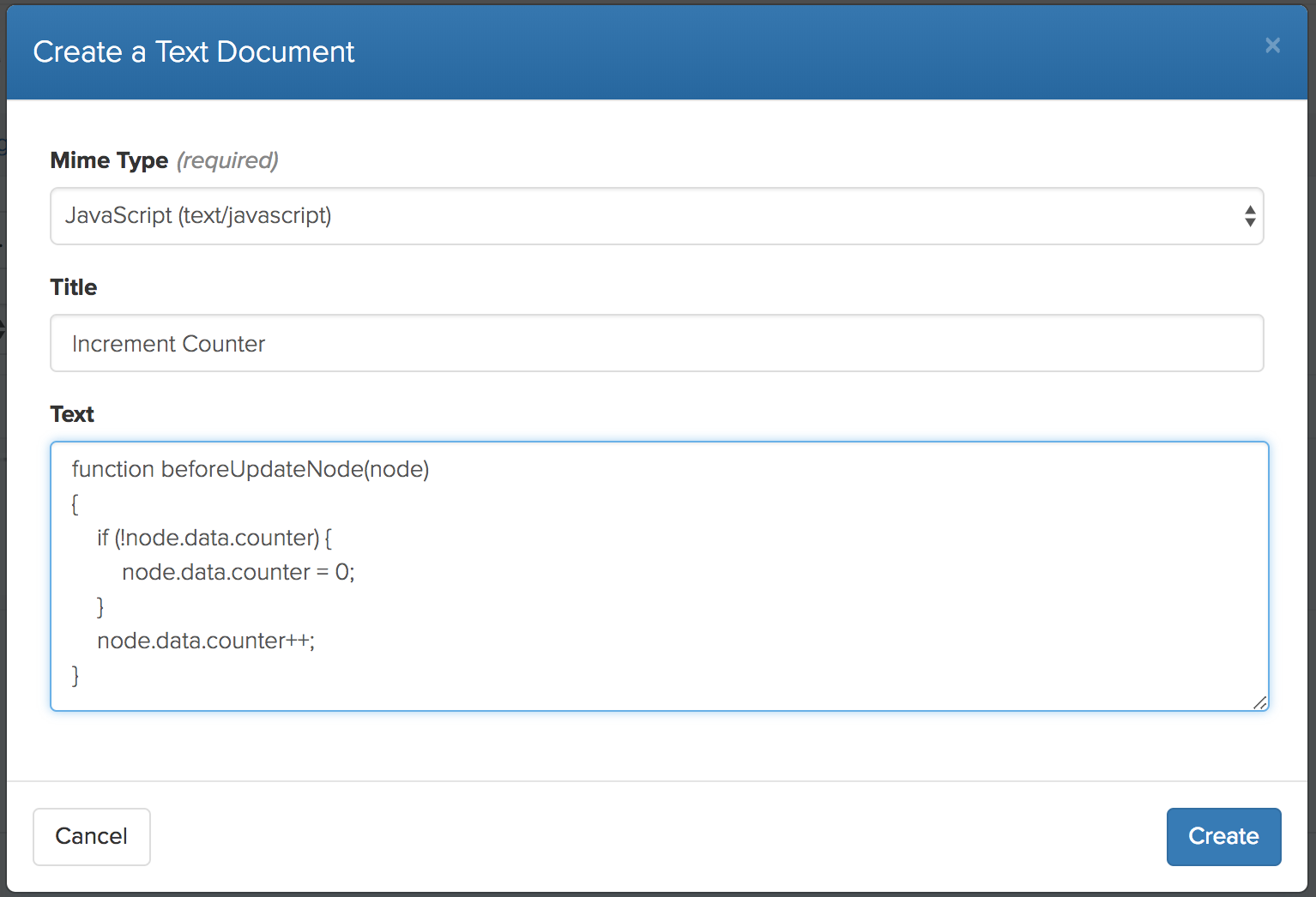
When the modal pops up, select text/javascript
for the file type and give the new text file the name Increment Counter
. Finally, fill in the the following JavaScript to implement the p:beforeUpdateNode
policy behavior:
function beforeUpdateNode(node)
{
if (!node.data.counter) {
node.data.counter = 0;
}
node.data.counter++;
}
Then click Save. Your script node is now created.
You can now inspect this script node if you want. By clicking into it, you can see it is a regular old n:node
content instance with a default
attachment of type text/javascript
.
If you wish to make changes to this code, you can click on the Editor page while viewing your document. You can make any changes you'd like and save those changes at any time.
This defines a handler for the p:beforeCreateNode
policy and uses the same method name (which is required). The method takes in the node being updated and makes sure there is a counter
key/value initialized to zero. It increments the counter each time it runs.
Now let's mark this JavaScript node as a behavior. That way, Cloud CMS knows how to work with it. Click on Features.
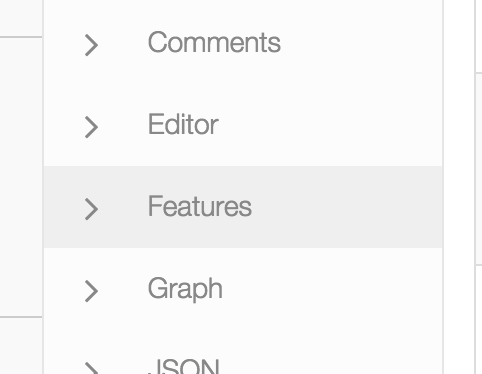
And then click on Add Feature. Pick the f:behavior
feature. In the JSON configuration for the feature, just set enabled
to true
. Like this:
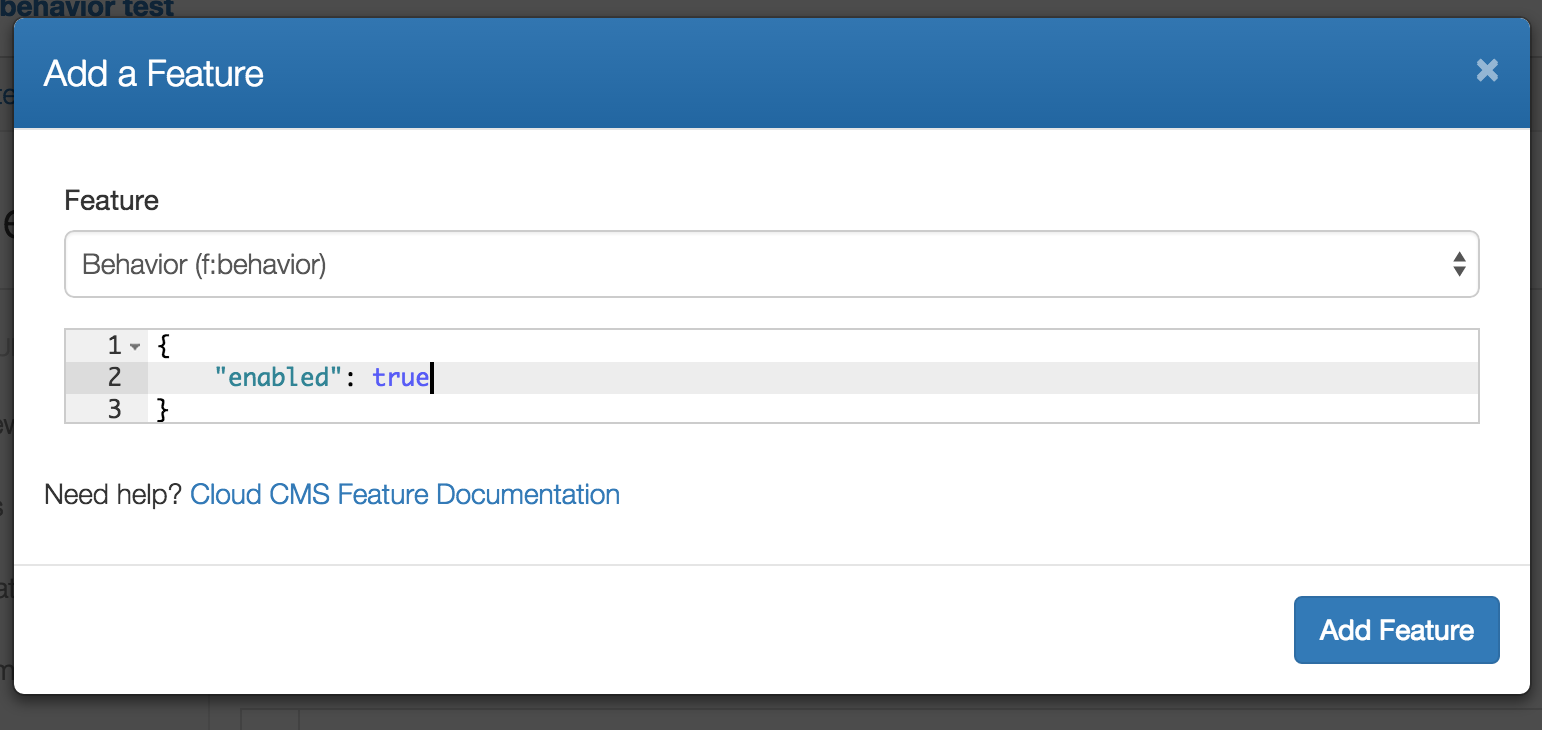
And then click Add Feature to save things.
Create the Article Type
Now let's create a simple content type for an article. We'll then bind our behavior to the content type so that all of the articles we create will automatically have this counter logic applied to them.
In your project in Cloud CMS and then go to the Content Model to create a new Content Type with QName custom:myArticle
.
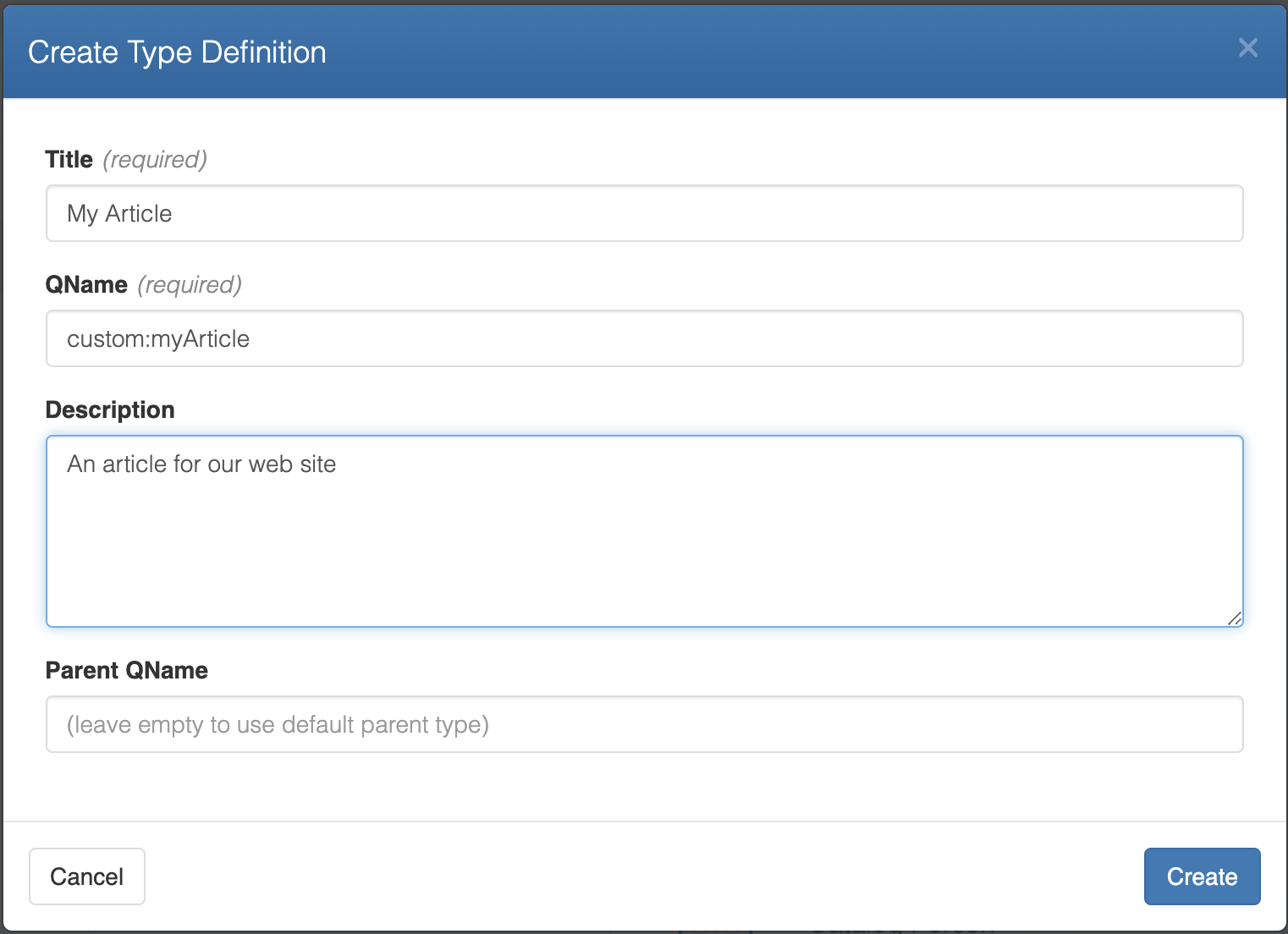
The Content Builder will open the article and you can edit usint he buider or edit the JSON. We can use JSON Schema here to define any properties that we like. Let's keep it simple since the point of this exercise isn't really to model out something cool (see the section on Content Modeling for that)! Rather, let's just define title and description, like this:
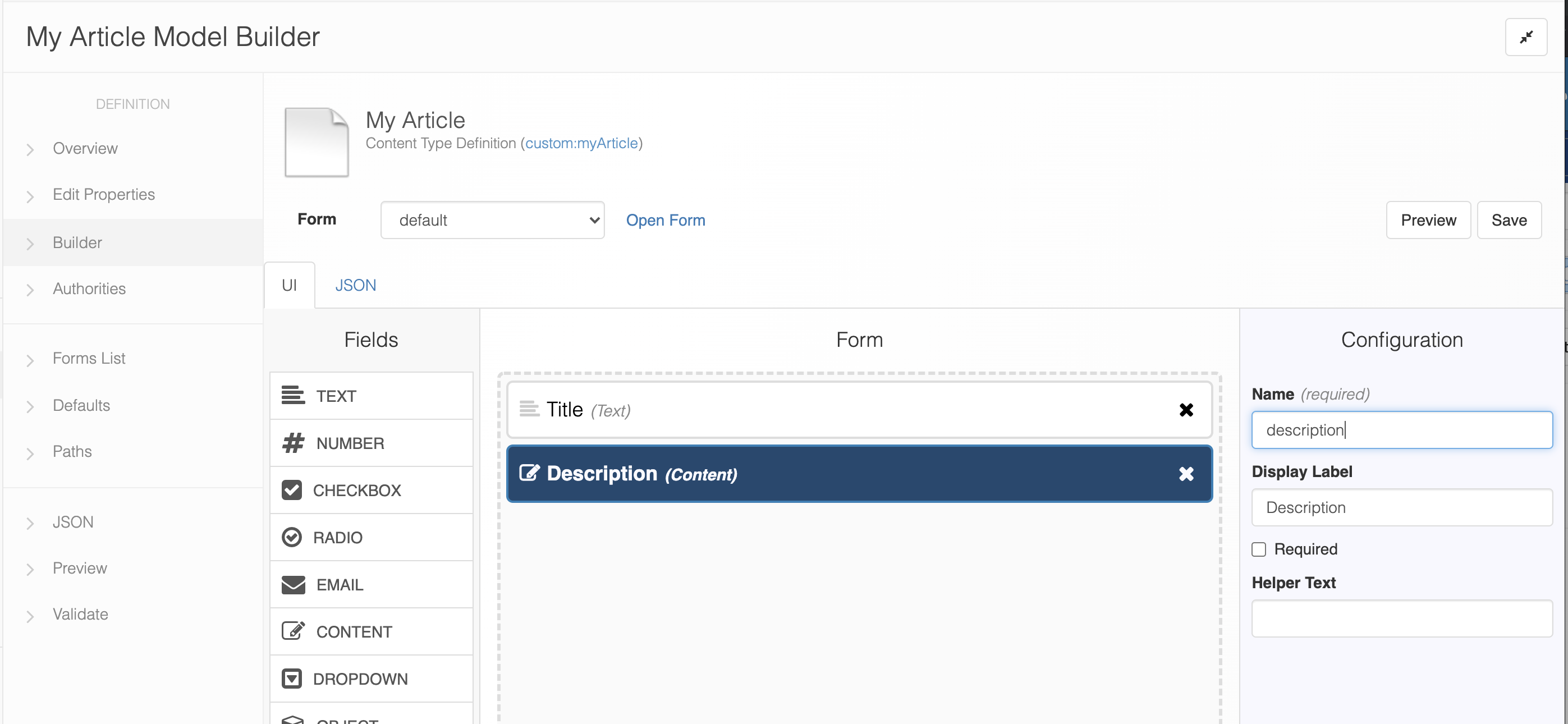
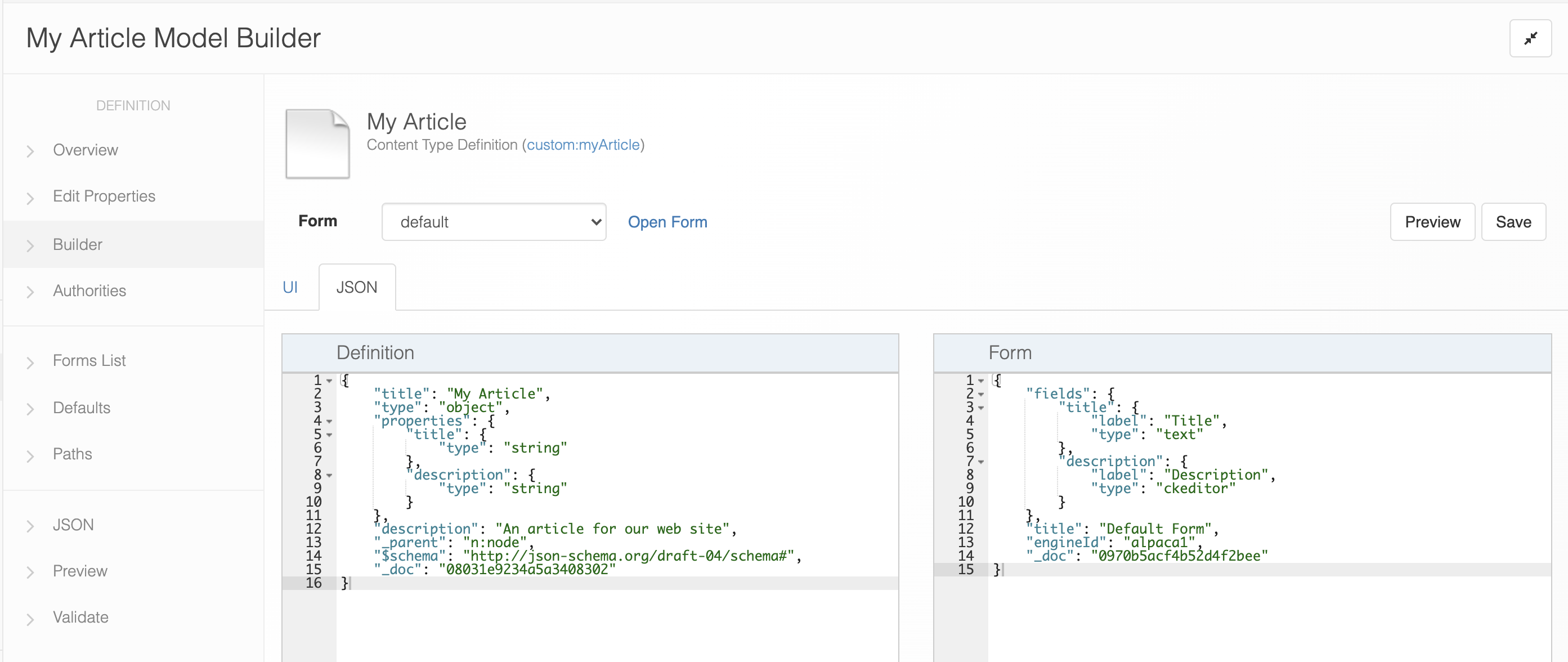
Be sure to save any changes to your content type.
Bind the Behavior to the Content Type
We're not done with the content type yet.
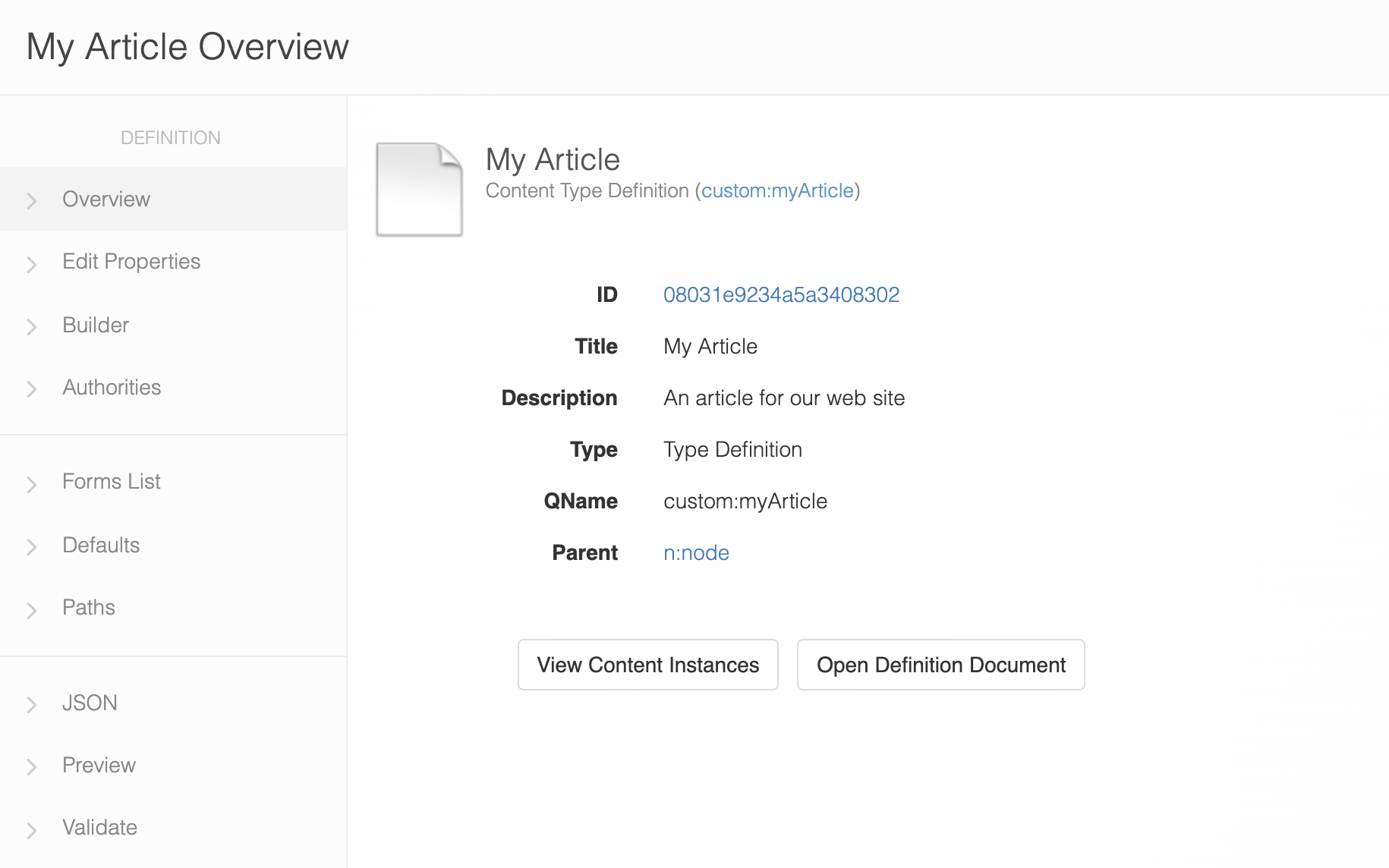
On the Overview page of the definition, click its ID and it takes you to the document view of the definition. Then click on Associations.
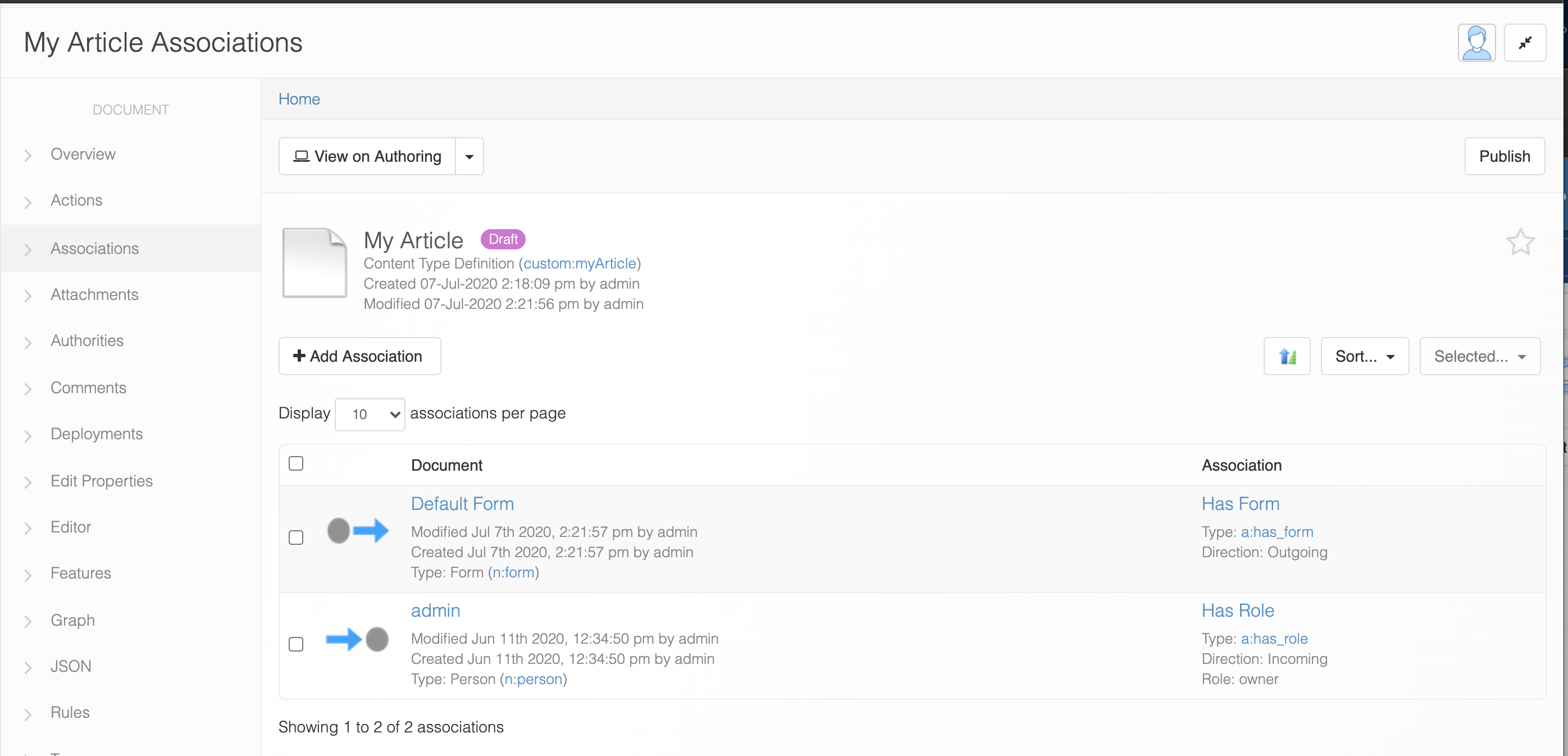
And then click on Add Association. Here, we create an association between our Content Type node and the Behavior node. The association should be of type Has Behavior (a:has_behavior
). Use the Select... button to find and pick your Increment Counter
behavior.
Furthermore, we need to supply some additional properties that are stored on the association, as shown here:
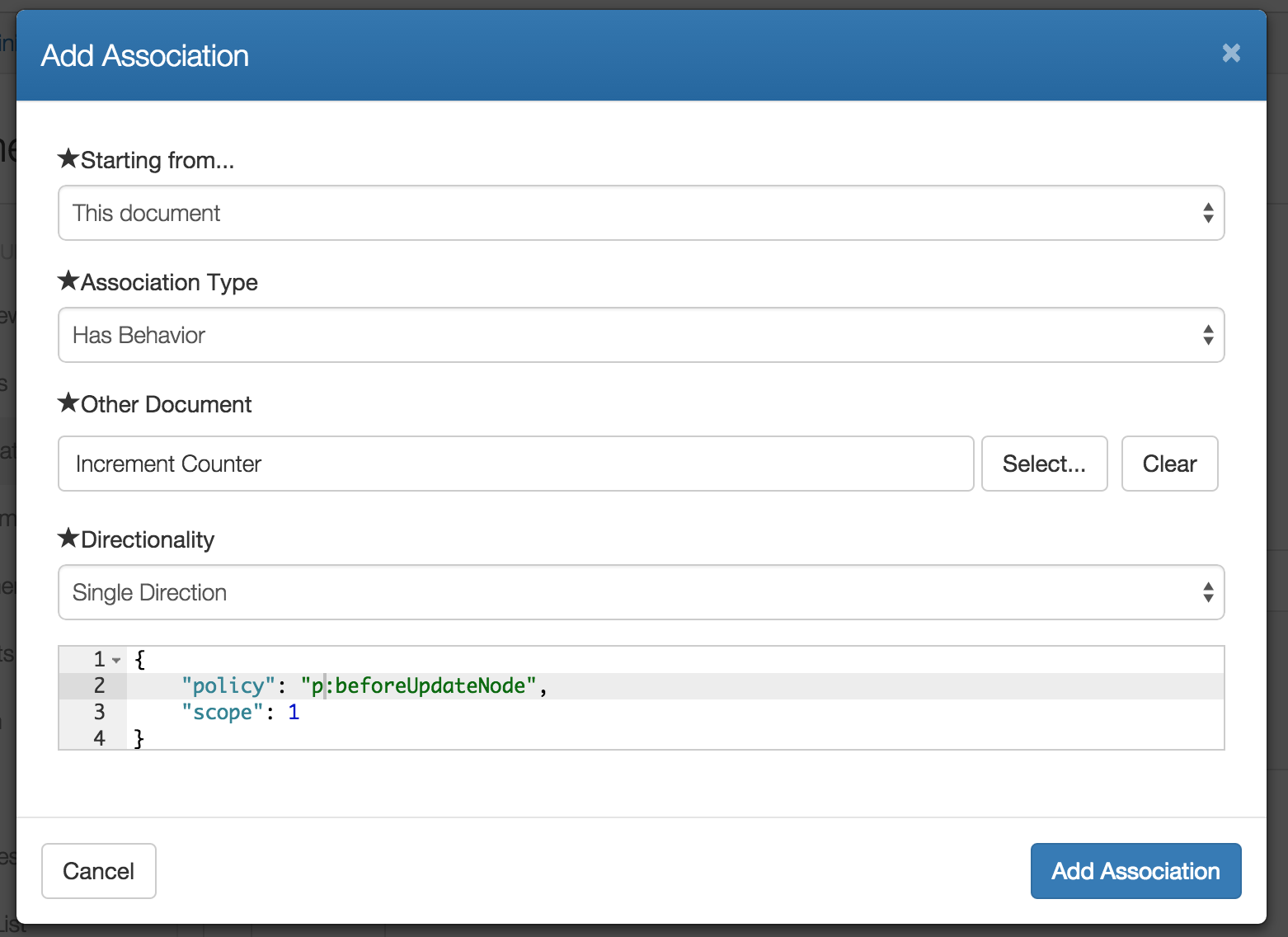
The additional properties are:
{
"policy": "p:beforeUpdateNode",
"scope": 1
}
The policy
property lets the association know which policy method is being overridden. The scope
property defines the intersection point for the policy method override. To override a content instance's behaviors, the value should be set to 0. To override all content instances for a content type, the value should be set to 1. Note that a value of 1 only applies to content type nodes.
Click Add Association to lock things into place.
Try it out
In the previous steps, we've overridden a behavior for all instances of a content type. Whenever any instance of the content type my:article
is updated, a counter will be maintained and incremented automatically.
To try it, go to Documents and create an article. To do so, click on Create a Document and pick the type My Article
.
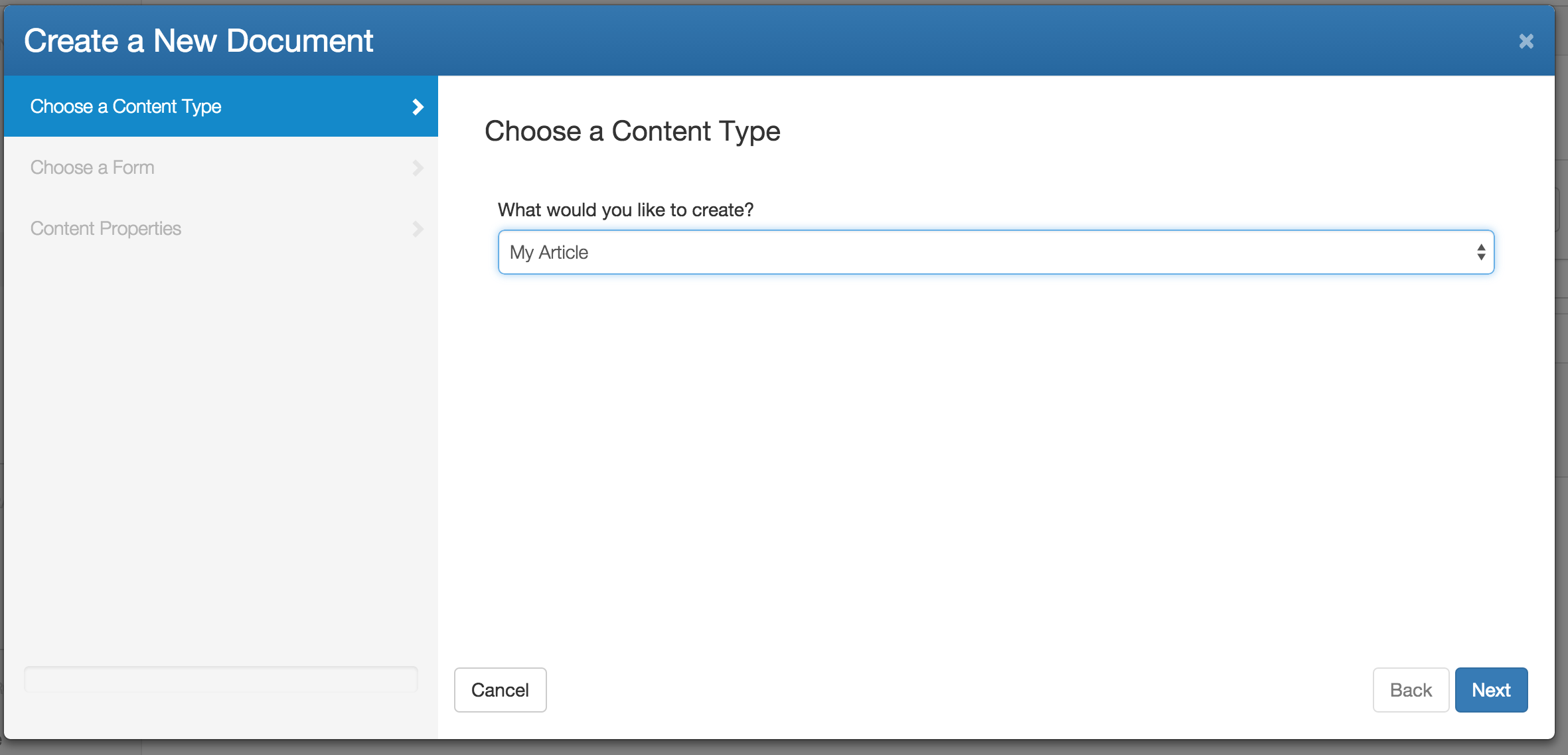
Enter anything you'd like for Title and Description and then click Create.
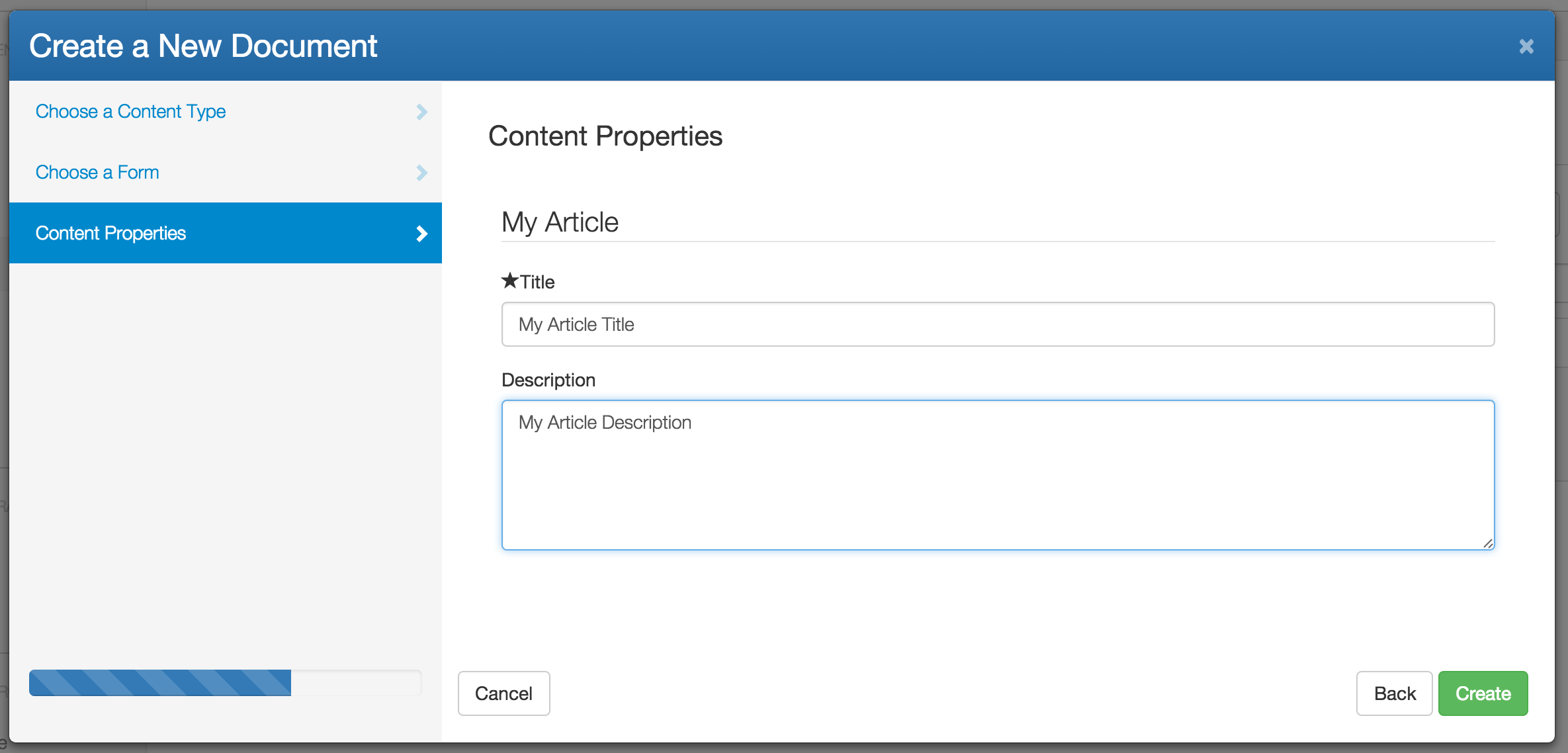
Now click on your Article to open up the overview screen. On the right-hand side, you'll see a Properties panel.
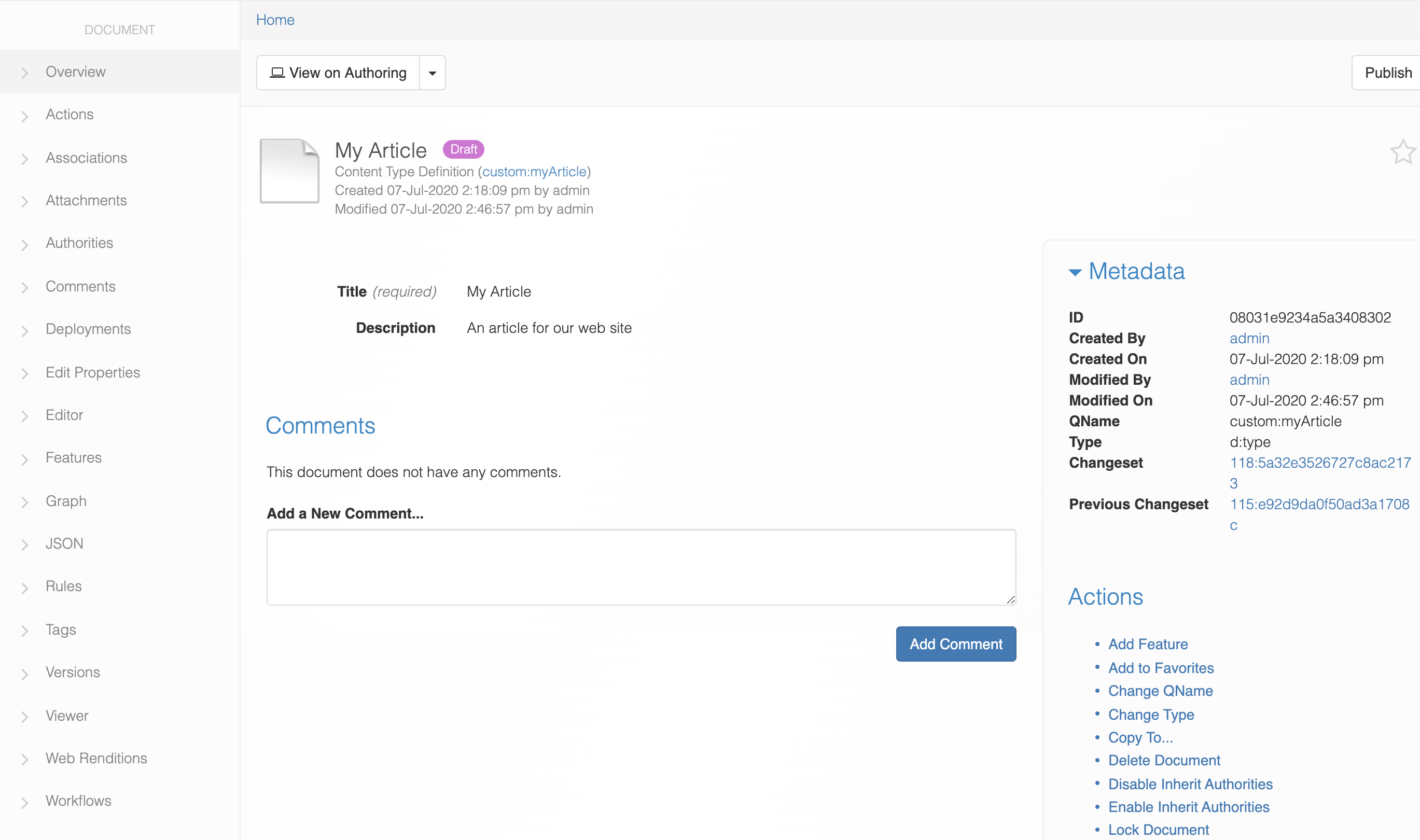
Same old standard properties, right? That's because we haven't updated our article yet. Remember that our policy override won't trigger until we do an update to the article. To update, click on Refresh Document
on the actions menu on the right-hand side.
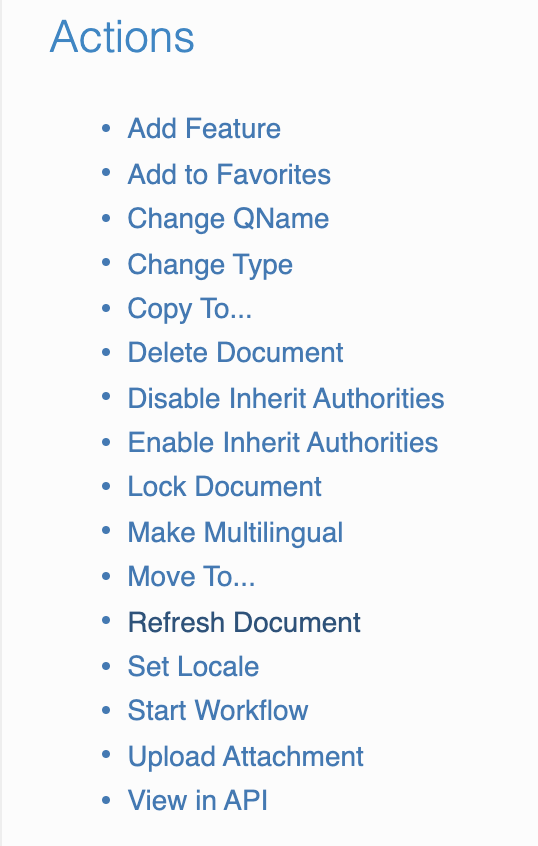
Your screen will refresh and you'll see that the counter
property has incremented to 1.
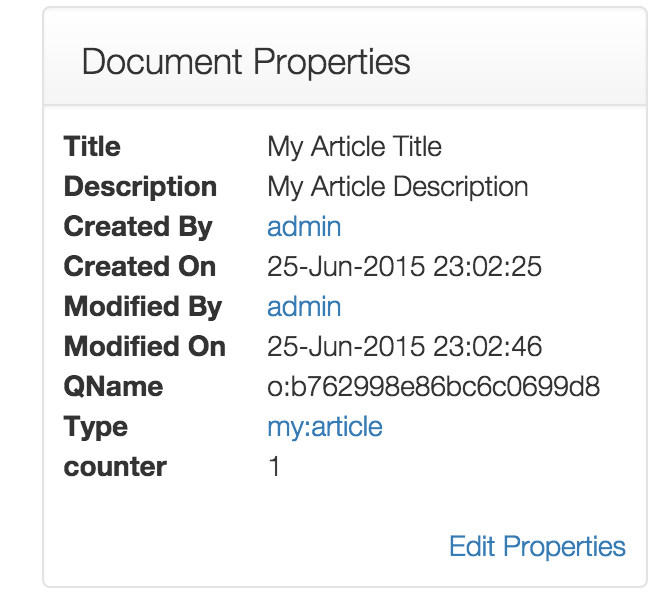
Click on Refresh Document
a few more times. Your counter will kindly increment for you.
That's it. Your behavior is in place and will run automatically for all update operations against any and all content instances of type my:article
.