Nodes
The Cloud CMS Bulk Import tool makes it easy to import content from structured data files stored on disk. The Packager API provides several methods which can read content from directories and bulk import that content all in one fell-swoop. This includes content types, content instances and relational information between content items.
This is useful for many scenarios including those where you may wish to store a master copy of your foundation content types and content instances for new projects.
Suppose we have a series of Articles that are written by two well-known authors, Daenerys Targaryen and Jon Snow.
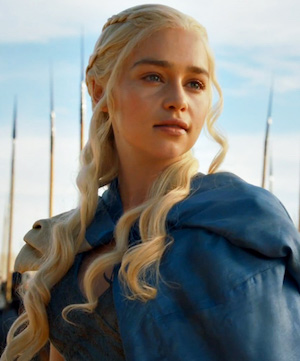
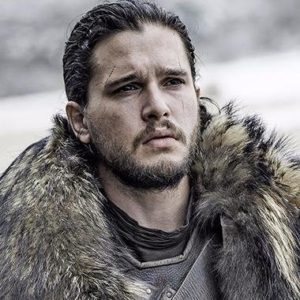
We define the following content types:
my:article
my:author
We also define an association type that relates Articles to Authors:
my:authored-by
The article content type definitions may be stored on disk and have relator properties that point to their author instances. The authoredBy
property is a relator property as shown here:
{
"title": "Article",
"type": "object",
"properties": {
"authoredBy": {
"title": "Authored By",
"type": "object",
"_relator": {
"nodeType": "my:author",
"associationType": "my:authored-by"
}
}
},
"_qname": "my:article",
"_type": "d:type"
}
Article instances in turn can use the special __related_node__
field to connect Articles to Authors during the import:
[{
"title": "My first article",
"body": "Body for first article",
"authoredBy": {
"__related_node__": "dt"
},
"_key": "article1"
}]
Where dt
is the _alias
for the Daenarys Targaryen author.
In total, the files on disk might look like this:
data\
articles.json
authors.json
daenerys_targaryen.jpg
jon_snow.jpg
types\
my_article\
node.json\
my_author\
node.json\
my_authored_by\
node.json\
We can use the following code to collect all this into our import package:
// create a packager
var PackagerFactory = require("cloudcms-packager");
PackagerFactory.create(function(err, packager) {
// package up content type definitions
packager.addFromDisk("./types/my_article/node.json");
packager.addFromDisk("./types/my_author/node.json");
packager.addFromDisk("./types/my_authored_by/node.json");
// package up articles and authors
packager.addFromDisk("./data/authors.json", "my:author");
packager.addFromDisk("./data/articles.json", "my:article");
// add attachments for Daenerys and Jon
packager.addAttachment("dt", "default", "./data/daenerys_targaryen.jpg");
packager.addAttachment("js", "default", "./data/jon_snow.jpg");
// commit
packager.package(function(err, archiveInfo) {
console.log("Wrote archive: " + archiveInfo.filename);
});
});
For a full example of this code in action, please see:
https://github.com/gitana/cloudcms-packager/tree/master/examples/nodes/example1