JavaScript (Legacy)
Connect your HTML browser applications to Gitana
Latest Version | |
---|---|
Version | undefined |
Date |
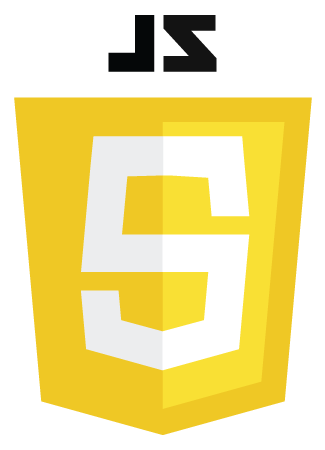
The Gitana JavaScript driver can be dropped into any web or mobile application running in the browser, within Node.js or any of a host of web frameworks including jQuery, Vue.js, Next.js, Nuxt and many others.
Loading the Driver
In the Browser
<a class='named-link' name='using-amd'></a>
### Using AMD
define(["gitana"], function(Gitana) { Gitana.connect({...}, function() { // ...your code }); });
<a class='named-link' name='using-common-js'></a>
### Using Common JS
var Gitana = require("gitana"); Gitana.connect({...}, function() { // ...your code });
<a class='named-link' name='installation'></a>
## Installation
You can get the Gitana JavaScript driver using Bower, NPM, via a direct download or you can load it
from the Cloud CMS CDN.
<a class='named-link' name='install-using-bower'></a>
### Install using Bower
bower install gitana
<a class='named-link' name='install-using-npm'></a>
### Install using NPM
npm install gitana
<a class='named-link' name='direct-download'></a>
### Direct Download
<ul class="normal">
<li>
<a target="_blank" href="${releaseUrl1}">gitana.js</a>
</li>
<li>
<a target="_blank" href="${releaseUrl2}">gitana.min.js</a>
</li>
</ul>
The Gitana driver comes wrapped with a shim to auto-detect the presence of an AMD and CommonJS loaders.
This allows it to be loaded into a segmented namespace via tools like RequireJS within the browser or Node.js
on the server-side. The driver is entirely standalone and has no external dependencies.
<a class='named-link' name='getting-started'></a>
## Getting Started
To connect to Gitana, you first need to have a set of API Keys. In Gitana, API Keys consists of
a client key/secret and a user key/secret. You pass these keys into the <code>Gitana.connect</code>
method as a JSON object.
You can create as many API Keys as you would like. The basic process for getting your own keys is
described in the <a href="/documentation/gitana/4.0/developers/apikeys.html">API Keys</a>. Give that a shot and
when you're done, you should have the following:
<ul>
<li><code>clientKey</code></li>
<li><code>clientSecret</code></li>
<li><code>username</code></li>
<li><code>password</code></li>
</ul>
You can then connect to Gitana using a code block like the one shown below. Make sure to plug in the
values for <code>clientKey</code>, <code>clientSecret</code>, <code>username</code> and <code>password</code>
from the API Keys that you generate.
Gitana.connect({ "clientKey": "{clientKey}", "clientSecret": "{clientSecret}", "username": "{username}", "password": "{password}", "baseURL": "https://api.cloudcms.com" }, function(err) {
// here is some sample code of what we might then do
// create a repository, get master branch
this.createRepository().readBranch("master").then(function() {
// create a few nodes
this.createNode();
this.createNode({ "title": "My second node" });
this.createNode({ "title": "My third node", "name": "boo" });
// query for nodes
this.queryNodes({ "name": "boo" }).count(function(count) {
console.log("count = " + count); // 1
});
});
// create then delete a domain
this.createDomain({
"title": "Users and Groups"
}).del();
});
<a class='named-link' name='documentation'></a>
## Documentation
The JavaScript driver is a very powerful library that provides access to 100% of the capabilities of
Gitana from a programmatic perspective. It is therefore important to first familiarize yourself
with the concepts presented in the <a href="/documentation.html">Gitana Documentation</a>.
<!-- We also provide <a href="https://code.cloudcms.com/gitana-javascript-driver/${releaseVersion}/jsdoc/index.html" target="_blank">JS-Doc Code Documentation</a> for all of the driver's classes and methods. -->
You should familiarize with the <a href="https://api.cloudcms.com/docs" >Gitana REST API</a> which covers HTTP-level
information about all of the methods and operations available within Gitana.
Check out our <a href="/documentation/gitana/4.0/developers/cookbooks/javascript.html">Gitana JavaScript Cookbook</a> for recipes and examples
using the JavaScript Driver.
<a class='named-link' name='fork-the-code'></a>
## Fork the Code
The Gitan JavaScript driver is 100% open-source (Apache 2.0) and so you're free to fork it, extend it and
dig into it to learn about how it works. We provide hundreds of test files and thousands of unit tests
within that demonstrate various code operations. It's really cool.
<ul>
<li><a target="_blank" href="https://github.com/gitana/gitana-javascript-driver">View the Source Code</a></li>
</ul>
<a class='named-link' name='bugs--problems'></a>
## Bugs / Problems
If you run into a bug, please <a href="https://github.com/gitana/gitana-javascript-driver/issues">create an issue</a>
so that we can look at it.
<br/>
For production support, please contact us at
<a href="mailto:support@gitana.io">support@gitana.io</a> or call us directly.
<a class='named-link' name='support-and-terms-of-use'></a>
## Support and Terms of Use
The JavaScript driver is free to use in your applications and projects. It is fully supported by Gitana.