Go
Connect your Go applications to Gitana
Latest Version | |
---|---|
Version | undefined |
Date |
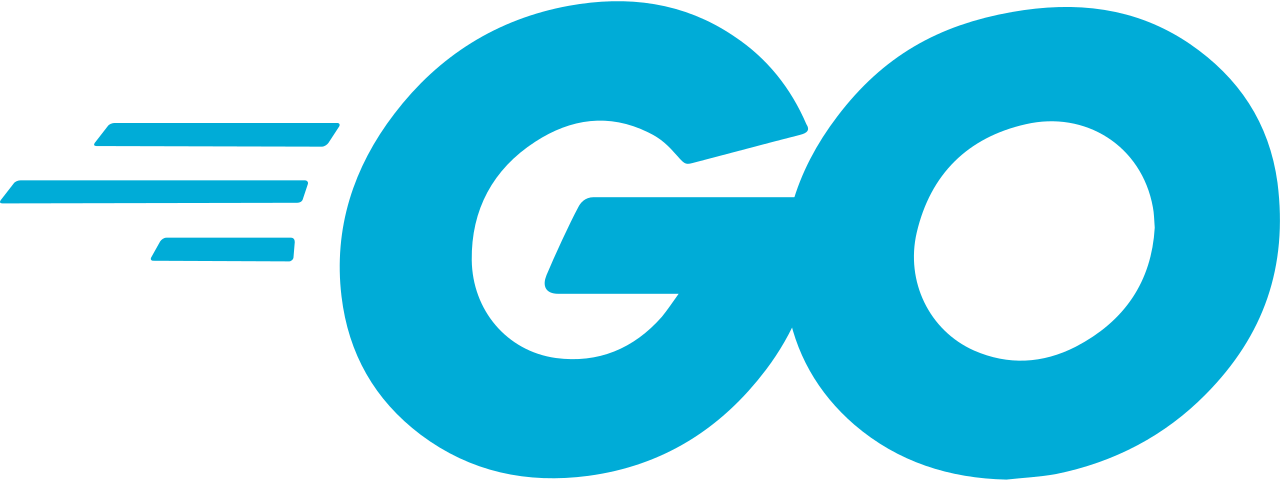
Current version: v0.1.3. Released Jun 13, 2022
The Gitana Go driver allows you to easily connect your Go applications to the Gitana server.
Currently supports the following functionality:
- Connect to and refresh access tokens with the API
- Read platform, branches, repositories, projects, releases
- Read, query, search, create, update, and delete nodes
- Manage node translations, versions, associations, attachments
- Make graphql queries
- And more!
Installation
go get github.com/gitana/cloudcms-go-driver
Connecting to Gitana
ConnectDefault
looks for a gitana.json
file in your working directory, where as Connect
allows you to pass the required authentication settings.
The required API key properties for this are:
clientKey
clientSecret
username
password
baseURL
You can also provide the flag debug: true
in your gitana.json
/ Gitana config object to provide additional request logging.
Connection Examples:
// Connect to Gitana using gitana.json in working directory
session, err := cloudcms.ConnectDefault()
if err != nil {
fmt.Println(err)
return
}]
session, err = cloudcms.Connect(&cloudcms.CloudcmsConfig{
Client_id: "clientId",
Client_secret: "clientSecret",
Username: "username",
Password: "password",
BaseURL: "baseURL",
})
Examples
Below are some examples of how you might use the driver:
package main
import (
"github.com/gitana/cloudcms-go-driver"
)
func main() {
// Connect to Gitana using gitana.json in working directory
session, err := cloudcms.ConnectDefault()
if err != nil {
fmt.Println(err)
return
}
var repositoryId string
// List branches
branches, _ := session.ListBranches(repositoryId, nil)
// Read branch
branchId := "master"
branch, _ := session.ReadBranch(repositoryId, branchId)
// Read Node
node, _ := session.ReadNode(repositoryId, branchId, nodeId)
// Create Node
nodeObj := cloudcms.JsonObject{
"title": "Twelfth Night",
"description": "An old play",
}
nodeId, _ := session.createNode(repositoryId, branchId, nodeObj, nil)
// Query Nodes
query := cloudcms.JsonObject{
"_type": "store:book",
}
pagination := cloudcms.JsonObject{
"limit": 1,
}
queriedNodes, _ session.QueryNodes(repositoryId, branchId, query, pagination)
// Find Nodes
find := cloudcms.JsonObject{
"search": "Shakespeare",
"query": JsonObject{
"_type": "store:book",
}
}
findNodes, _ := session.FindNodes(repositoryId, branchId, find ,nil)
}
Documentation
It is therefore important to first familiarize yourself with the concepts presented in the Gitana Documentation
Check out our Gitana Go Cookbook for recipes and examples using the Go Driver.
Fork the Code
Bugs / Problems
For production support, please contact us at support@gitana.io or call us directly.