C# Driver
Connect your .NET applications to Gitana
Latest Version | |
---|---|
Version | undefined |
Date |
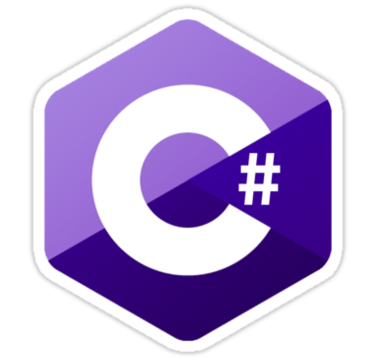
The Gitana C# driver allows you to easily connect your C# and .NET applications to the Gitana server.
Currently supports the following functionality:
- Connect to and refresh access tokens with the API
- Read platform, branch, and repositories
- Read, query, search, create, update, and delete nodes
Installation
Install this driver as you would any other NuGet package. Command Line:
dotnet add package cloudcms
Visual Studio:
Install-Package cloudcms
URL:
Connecting to Gitana
To connect to Gitana, use the static CloudCMSDriver.ConnectAsync
method. This takes either a file path to a gitana.json
file, a JObject json object, dictionary, or ConnectionObject.
The required API key properties for this are:
clientKey
clientSecret
username
password
baseURL
Connection Examples:
string path = "gitana.json";
IPlatform platform1 = await CloudCMSDriver.ConnectAsync(path);
JObject configObj = ...;
IPlatform platform2 = await CloudCMSDriver.ConnectAsync(configObj);
IDictionary<string, string> configDict = ...;
IPlatform platform3 = await CloudCMSDriver.ConnectAsync(configDict);
ConnectionConfig config = ...;
IPlatform platform4 = await CloudCMSDriver.ConnectAsync(config);
</pre>
<h2>Examples</h2>
<p>Below are some examples of how you might use the driver:</p>
<pre class="prettyprint linenums lang-csharp">
// Connect to Cloud CMS
string path = "gitana.json";
IPlatform platform = await CloudCMSDriver.ConnectAsync(path);
// Read repository
IRepository repository = await platform.ReadRepositoryAsync("<repositoryId>");
// Read branch
IBranch branch = await repository.ReadBranchAsync("<branchId>");
// Read node
INode node = await branch.ReadNodeAsync("<nodeID>");
// Update node
node.Data["title"] = "A new title";
await node.UpdateAsync();
// Delete node
await node.DeleteAsync();
// Create node
JObject obj = new JObject(
new JProperty("title", "Twelfth Night"),
new JProperty("description", "An old play")
);
INode newNode = await branch.CreateNodeAsync(obj);
// Query nodes
JObject query = new JObject(
new JProperty("_type", "store:book")
);
JObject pagination = new JObject(
new JProperty("limit", 2)
);
List<INode> queryNodes = await branch.QueryNodesAsync(query, pagination);
// Search/Find nodes
JObject find = new JObject(
new JProperty("search", "Shakespeare"),
new JProperty("query",
new JObject(
new JProperty("_type", "store:book")
)
)
);
List<INode> findNodes = await branch.FindNodesAsync(find, pagination);
Documentation
The C# driver is a useful library that provides access to the capabilities of Gitana from a programmatic perspective. It is therefore important to first familiarize yourself with the concepts presented in the Gitana Documentation.
Check out our Gitana C# Cookbook for recipes and examples using the C# Driver.
Fork the Code
The Gitana C# driver is 100% open-source (Apache 2.0) and so you're free to fork it, extend it and dig into it to learn about how it works.
Bugs / Problems
If you run into a bug, please create an issue so that we can look at it.
For production support, please contact us at support@gitana.io or call us directly.
Support and Terms of Use
The C# driver is free to use in your applications and projects. It is fully supported by Gitana.