Java
Connect your Java, Spring MVC and Android applications to Gitana
Latest Version | |
---|---|
Version | undefined |
Date |
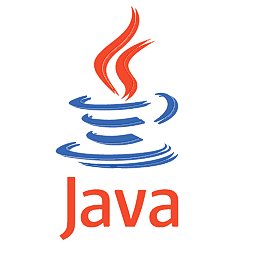
The Gitana Java driver makes it easy for your Java and Android applications to connect to and communicate with the Gitana server. The driver can be utilized with Android mobile devices as well as Java applications running on the server or as a web application (Spring Framework, SEAM, etc, running on Tomcat, Glassfish, WebSphere and many other frameworks).
Maven Repository
Gitana runs a Maven repository where it hosts its Java-related assets including the Gitana driver. If you're building using Maven, Ivy, Gradle or another build framework that manages dependencies, you can probably take advantage of this repository.
For Maven users, you can add the following repository configuration:
<repositories>
<repository>
<id>cloudcms-public</id>
<name>cloudcms-public</name>
<url>https://maven.cloudcms.com/public</url>
</repository>
</repositories>
You can then add the Java Driver as a dependency to your pom.xml file:
<dependencies>
<dependency>
<groupId>org.gitana</groupId>
<artifactId>gitana-java-driver</artifactId>
<version></version>
</dependency>
</dependencies>
Getting Started
To connect to Gitana, you first need to have a set of API Keys. In Gitana, API Keys consists of a client key/secret and a user key/secret. You pass these keys into the Gitana.connect
method as a JSON object.
You can create as many API Keys as you would like. The basic process for getting your own keys is described in the API Keys. Give that a shot and when you're done, you should have the following:
clientKey
clientSecret
username
password
You can then connect to Gitana using a code block like the one shown below. Make sure to plug in the values for clientKey
, clientSecret
, username
and password
from the API Keys that you generate.
package com.cloudcms;
import org.gitana.platform.client.branch.Branch;
import org.gitana.platform.client.datastore.DataStore;
import org.gitana.platform.client.node.BaseNode;
import org.gitana.platform.client.node.Node;
import org.gitana.platform.client.platform.Platform;
import org.gitana.platform.client.platform.PlatformDataStore;
import org.gitana.platform.client.project.Project;
import org.gitana.platform.client.repository.Repository;
import org.gitana.platform.support.Pagination;
import org.gitana.platform.support.QueryBuilder;
import org.gitana.platform.support.ResultMap;
import org.gitana.platform.client.Gitana;
import java.util.List;
public class CloudCMSExample {
public static void main(String[] args) {
// these values come from your API Keys
String clientKey = "{clientKey}";
String clientSecret = "{clientSecret}";
String username = "{username}";
String password = "{password}";
// connect to Cloud CMS as your application user
Gitana gitana = new Gitana(clientKey, clientSecret);
Platform platform = gitana.authenticate(username, password);
// find my project
Project myProject = null;
List<Project> projectList = platform.listProjects().asList();
for (Project project : projectList) {
if (project.getTitle().equalsIgnoreCase("My Project Title")) {
myProject = project;
break;
}
}
// there are a few data stores in each project. we want the 'Content' repository
Repository myRepository = null;
List<PlatformDataStore> datastores = myProject.getStack().listDataStores().asList();
for (DataStore dataStore : datastores) {
if (dataStore.getTypeId().equalsIgnoreCase("repository")) {
// this is the content repository for this project
myRepository = (Repository)dataStore;
break;
}
}
// get the master branch
Branch master = myRepository.readBranch("master");
Node node1 = (Node) master.createNode();
Node node2 = (Node) master.createNode();
Node node3 = (Node) master.createNode();
// update a node
node2.set("axl", "rose");
node2.update();
// delete the third node
node3.delete();
// query for nodes
ResultMap<BaseNode> nodes = master.queryNodes(QueryBuilder.start("axl").is("rose").get(), new Pagination(0,2));
for (BaseNode node: nodes.values()) {
System.out.println("id: " + node.getId());
System.out.println("axl: " + node.get("axl"));
}
}
}
Documentation
The Java driver is a useful library that provides access to the capabilities of Gitana from a programmatic perspective. It is therefore important to first familiarize yourself with the concepts presented in the Gitana Documentation.
You should also familiarize with the Gitana REST API which covers HTTP-level information about all of the methods and operations available within Gitana.
Check out our Gitana Java Cookbook for recipes and examples using the Java Driver.
Fork the Code
The Gitana Java driver is 100% open-source (Apache 2.0) and so you're free to fork it, extend it and dig into it to learn about how it works. We provide hundreds of test files and thousands of unit tests within that demonstrate various code operations. It's really cool.
Bugs / Problems
If you run into a bug, please create an issue so that we can look at it.
For production support, please contact us at support@gitana.io or call us directly.
Support and Terms of Use
The Java driver is free to use in your applications and projects. It is fully supported by Gitana.